Understanding CORS errors: Key causes and effective solutions
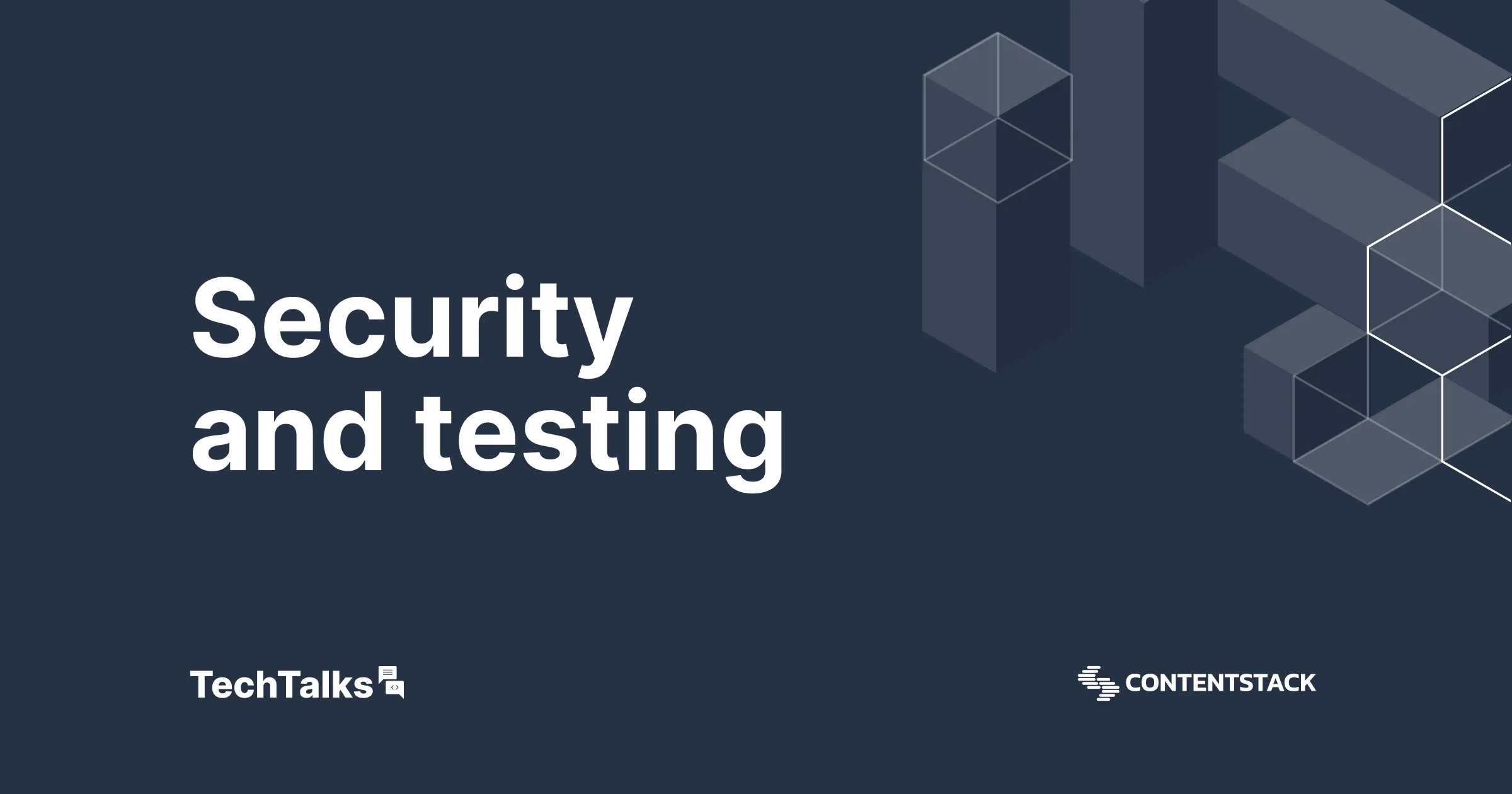
Understanding CORS errors is crucial for web developers. This blog explores the causes of CORS errors and offers practical solutions to ensure secure cross-origin resource sharing. Learn how to diagnose and fix CORS errors for a better web application's performance.
Highlights
You’ll learn about the causes of CORS error and its solutions.
Common Causes:
- Misconfigured CORS headers: Incorrect headers lead to denied access
- Preflight request failures: The server denies preflight requests due to misconfiguration
- Different origins: Mismatched client-server origins also trigger CORS errors
- Invalid or missing API keys: The server denies access without API keys, leading to a CORS error
Solutions:
- Adjust server-side settings to include correct CORS headers
- Use simple requests or match preflight requirements
- Verify API documentation for proper configuration
Fix your CORS errors to enjoy seamless web development. Switch to a compliant CMS. Talk to us today.
Keep reading to learn more!
Developers have asked over 14,600 questions relating to CORS errors on StackOverflow, which shows their importance in web development. CORS errors are an ever-present reality for web developers.
When you start building a web application, you may make link spelling mistakes or try to retrieve data from another server. That will throw up a CORS error since you are attempting to access resources from a different domain. CORS are essential for security. They prevent unauthorized access to resources from another domain without permission.
Developers must understand CORS errors, why they happen and how they work to enable them to apply the right fix.
What is a CORS error?
A CORS error is a security feature that occurs when a web application tries to access data in another domain without the right permissions. Here is how it works: If a website makes an API request to another website, the browser will get a CORS error, provided it has no permission.
Before CORS adoption, developers could only make API requests within their domain. However, they created CORS to enable websites to access resources in external domains without compromising on web security.
Common causes of CORS errors
So, what causes CORS error? Here are the most common causes of CORS errors:
- Misconfigured CORS headers
- Preflight request failures
- Different origins between client and server
- Invalid or missing API keys
Misconfigured CORS headers
Cross-origin request headers are like permission notes that show who can access resources and under what conditions. When these headers are not properly configured, the system spits out a CORS error.
To developers, a header is like a search warrant in the hands of law enforcement trying to access a property. Without it, there is no way to get in legally. Here are some important headers.
- Access-control-allow-origin headers: This header states that websites have the right permission to access resources.
- Access-control-allow-headers: These headers specify the headers you can include in the request as a security measure to confirm users.
- Access-control-allow-methods headers: These headers outline how you can access data, whether by PUT, GET, POST, or PUT.
Preflight request failures
If a preflight request fails, there will be a CORS error. But what is a preflight request?
A preflight request comes right before a website tries to access data from another online server. It asks the server for permission, and if it is declined, the preflight request fails. Incorrect server setup or configuration issues can cause preflight request failure.
Different origins between client and server
The “Access-control-allow-origin” header specifies the origins with permission to access APIs. If the requesting domain does not match the specified origin (port, scheme, or host), the browser turns down the request and gives a CORS error.
Invalid or missing API keys
API keys are secret codes used to authorize websites. Websites include them in headers when accessing another server. If the API key is wrong or missing, the server denies the request, and you will get a CORS error.
Overcome traditional CMS issues with Contentstack: Are you tired of slow development times and rising costs due to legacy monolithic suites? Contentstack offers a modern, component-based solution designed for the needs of today's enterprises. Discover agility and improved ROI. Request a demo to learn more.
How to fix CORS errors
Developers must be proactive in dealing with CORS errors. Here are practical ways they can do it.
Adjust server-side settings
Wrongly set headers will trigger a CORS error. So, include relevant CORS headers in your server responses to avoid that. These headers are:
- Access-control-allow-origin: Include the Access-control-allow-origin in server-side response headers.
- Access-control-allow-methods: Access-control-allow-methods include GET, POST, and PUT. It specifies allowed HTTP methods for cross-origin requests.
- Access-control-allow-headers: Use content-type or authorization. This header specifies allowed custom headers, ensuring only authorized headers are accepted for security
- Access-control-allow-credentials: The Access-control-allow-credentials response header tells web browsers whether the server allows cross-origin HTTP requests to include credentials. To give permission, set the withCredentials property on the XMLHttpRequest to true.
Client-side solutions
Not every request requires preflight requests. For instance, simple GET requests with no custom headers do not. However, if you cannot avoid preflight requests, ensure that the requests of your Fetch API conform with the server rules for headers and authorization tokens.
Fixing CORS errors in a web application
Imagine you want to fix CORS errors in a weather dashboard web application that shows real-time weather data. This kind of app will get real-time data from a weather API.
Debugging Process:
- Check the error: To start, check your developer console to know what CORS error you are dealing with. That lets you know what needs fixing, be it a missing header or unauthorized access.
- Review API documentation: Check the documentation for the weather API, which is usually available online. The API will indicate if CORS is enabled and any requirements for accessing data, such as allowed origins and required headers.
- Review request methods: Avoid custom headers unless necessary. Check that your Fetch API request uses a standard method like GET. Some APIs may not require preflight checks for these methods.
- Inspect preflight request, if applicable: If the API requires a preflight request, check the error message for details on missing or incorrect headers.
Depending on the API configuration, there are two methods to fix this error, as follows:
- Simple requests—no preflight: Check API documentation to confirm CORS is enabled for simple requests, such as GET and POST without custom headers. Focus on using standard methods in your Fetch API calls.
- Requests with preflight: If the API requires preflight checks, you must ensure your Fetch API request has the headers listed in the API documentation.
Here is an example using Fetch API with an Authorization header required by the server:
JavaScript
fetch('https://weatherapi.example.com/data/current', {
method: 'GET',
headers: {
'Authorization': 'Bearer YOUR_API_KEY'
}
})
.then(response => response.json())
.then(data => {
// Use the weather data here
})
.catch(error => {
console.error('Error fetching weather data:', error);
});
Always note that client-side solutions can help avoid CORS errors, but they cannot force a server to allow access if its CORS configuration is restrictive.
Troubleshooting CORS configuration problems
The most logical approach to solving any problem is understanding its root cause. This is a practical step-by-step approach to debugging CORS errors:
- Check your browser console: Open the developer console and look for CORS error messages. That will point you to the source of the problem, be it server or client.
- Check server response headers: If the error message points to server issues, take another look at the failed request's response headers. Look for the Access-control-allow-origin header. It should match your website's origin or be * for public access.
- Verify client-side request: If the error points to a client-side problem, verify that your Fetch API request code is correct. Use standard methods, like GET and POST and avoid unnecessary custom headers unless the API requires them.
- Match preflight requirements: If the source of the error is a preflight request, include server-specified headers like authorization tokens in your request.
Tools and techniques for diagnosing CORS Issues
You can diagnose CORS errors using several tools, such as Chrome’s DevTools, Postman and browser extensions like CORS helper. These tools allow you to inspect network requests and identify errors.
You can also check server headers to ensure they have the right configuration for Access-control-allow-origin. These tools allow you to streamline troubleshooting, ensuring safe cross-origin resource sharing.
Resolving CORS issues in web development
Troubleshooting CORS errors will resolve the issue, but taking a more proactive approach and preventing them is even better. A good CORS policy helps you achieve that. It allows you to prevent errors by specifying domains that can access your APIs.
Using proxy servers, You can adjust the web browser’s settings during development or route requests. These measures allow you to secure cross-origin resource sharing while maintaining the functions of your web app.
Contentstack: A Leader in CMS Performance. Experience the strength of Contentstack, a standout performer in Forrester's Q3 2023 CMS report. Contentstack simplifies your digital experience with our back-end extensibility and global deployments. Request a demo to learn more.
Case studies
Taxfix
Taxfix experienced issues with scalability and security and reached out to Contentstack. Contentstack’s headless CMS allowed them to upgrade their security and power over 300 content variables.
Teuber said. “We can use the same logic, we have all the components, the structure, the headers, it’s all way more scalable. I remember the designers were a little skeptical at the beginning, but now they are huge fans!”
Read more to see how Taxfix improved security with Contentstack’s headless CMS.
Health Karma
Health Karma needed a modular approach to manage security and chose Contentstack to deliver it.
The Contentstack headless CMS allowed them to control data access and improve security for their developers and web applications.
Michael Swartz had this to say.“The more we build in Contentstack, the more personalization we can offer, and the more scalable deployments we can do.”
Read more about Health Karma’s content scale-up after opting for a secure CMS.
FAQ section
How do you fix a CORS error?
To apply the right fix, you must first understand the cause of the error. Start by debugging the system. You may need to configure the server or use a browser extension or proxy to fix a CORS error.
What causes the CORS issue?
A CORS issue occurs when a web app requests resources from other systems without the right permissions. This triggers browser security policies.
What does CORS stand for?
CORS stands for cross-origin resource sharing (CORS). It allows browsers to restrict access to resources on its domain when a website tries to access sensitive data from another server.
What is the full form of a CORS error?
CORS is the short form for cross-origin resource sharing error.
Learn more
CORS is an essential security feature for modern web applications. It allows developers to access resources hosted on an external domain using APIs. Configure your server settings to specify allowed origins. Also, it should ensure that access is granted to specific methods and headers.
Contentstack allows you to manage CORS errors via a serverless function via custom extensions using UI extension SDK. Switch to a headless CMS with a robust CORS policy. Talk to us today!
About Contentstack
The Contentstack team comprises highly skilled professionals specializing in product marketing, customer acquisition and retention, and digital marketing strategy. With extensive experience holding senior positions in notable technology companies across various sectors, they bring diverse backgrounds and deep industry knowledge to deliver impactful solutions.
Contentstack stands out in the composable DXP and Headless CMS markets with an impressive track record of 87 G2 user awards, 6 analyst recognitions, and 3 industry accolades, showcasing its robust market presence and user satisfaction.
Check out our case studies to see why industry-leading companies trust Contentstack.
Experience the power of Contentstack's award-winning platform by scheduling a demo, starting a free trial, or joining a small group demo today.
Follow Contentstack on Linkedin
